What is Angular Lifecycle?
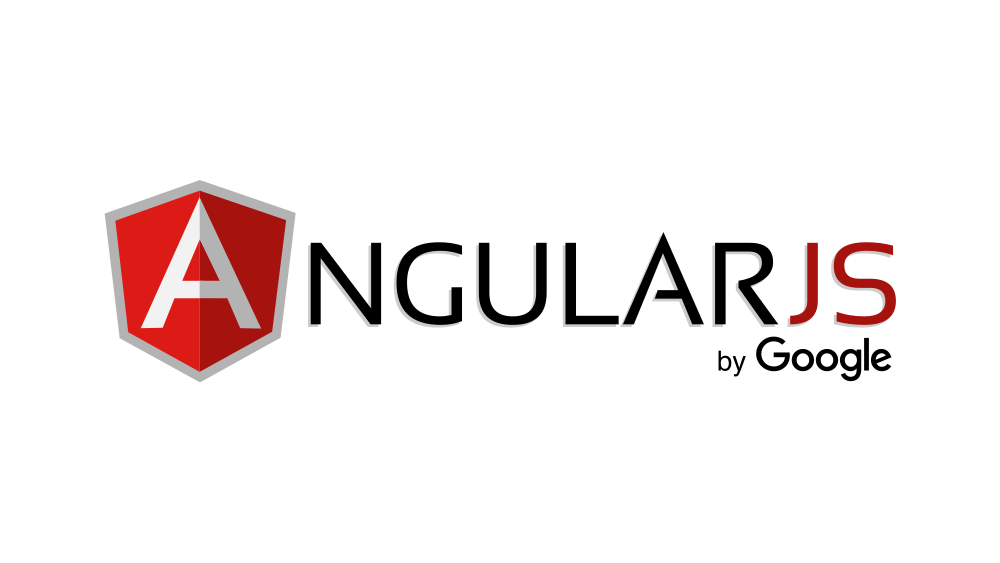
In Angular, components are the primary building block for any application. Every component has a lifecycle, process of creation, and executes all the functions. It was created to execute, then destroys instances in the course of execution.
There are 8 different stages within the component lifecycle. This is called a lifecycle hook event. We can use this event in different phases in any application to control the components.
Every component must have a constructor method. The constructor is executed first before the execution of other lifecycle events. A Component is a typescript class.
With support for different subjects in Angular like Behaviour subject, async subject, etc., it also has many amazing features and just because of that reason, it is the exceedingly recommended frontend framework by the software experts.
Talking about the components in Angular, here is the lifecycle sequence available in order in which they are invoked.
- ngOnChanges()
- ngOnInit()
- ngDoCheck()
- ngAfterContentInit()
- ngAfterContentChecked()
- ngAfterViewInit()
- ngAfterViewChecked()
- ngOnDestroy()
How to use Lifecycle event?
There are three steps to use the lifecycle event in angular:
Step 1: Import lifecycle event interface
Step 2: Declare component that implements lifecycle event interface
Step 3: Create the lifecycle event method
Let’s create a simple component, which implements the ngOnInit event.
Step 1: Import lifecycle event interface
You can import the lifecycle event from the core module. The name of the event is written in without ng. For example, the ngOnInit event is OnInit.

Step 2: Component implements lifecycle event interface
In AppComponent, implements the OnInit interface.

Step 3: Create a lifecycle event method
The method name has the same name as the lifecycle event.

ngOnChanges() :
- This event executes every time when the value of an input control within the component has been changed. Using this event, a parent component can communicate with its child component if the property decorator exposes @Input of the child component.
- If your component has not declared input or you can use without providing any inputs, ngOnChanges event is not called. To use the ngOnChanges() event, first we need to import OnChanges from the @angular/core library.
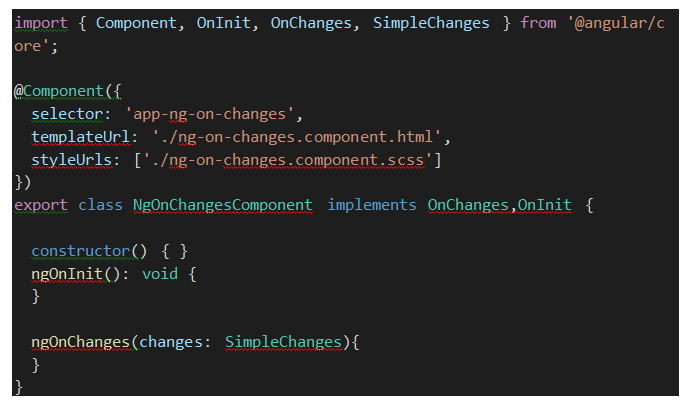
- ngOnChanges() event takes an object, that maps changed property named SimpleChange object, which takes the current and previous property value. For example, if two input property input1 and input2 are changed, the SimpleChanges object looks like this:
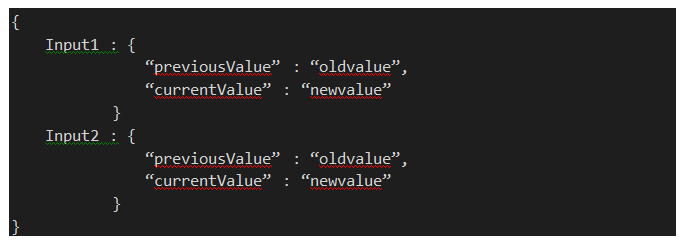
ngOnInit() :
- ngOnInit() method is called only once when the component is created for the first time. This event is called after ngOnChanges event. In this event, you can add the initialization logic for the component.
- To use the ngOnInit(), first we need to import OnInit from @angular/core library. Let’s see a simple example of ngOnInit().
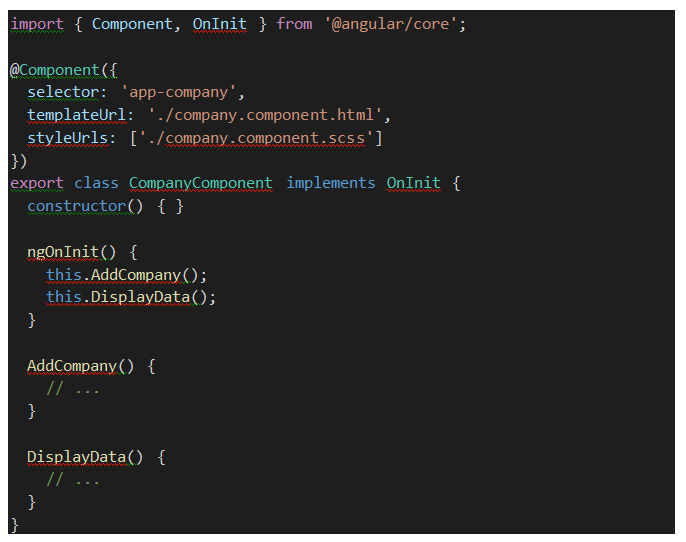
Difference between Constructor andngOnInit
The constructor is executed when the class is initialized. It is the feature of JavaScript. The ngOnInit() is an angular lifecycle event and is called component initialization with the input properties. The input properties are available below the ngOnInit lifecycle event. You should use the constructor to initialize the class members. ngOnInit is used for all the declaration and initialization.
ngDoCheck() :
- ngDoCheck() event is the third lifecycle event that gets called on a component. It invokes a custom change detection function logic for any component. When an input property of a component is checked then this event is triggered. Let’s see a simple example of the ngDoCheck() event.
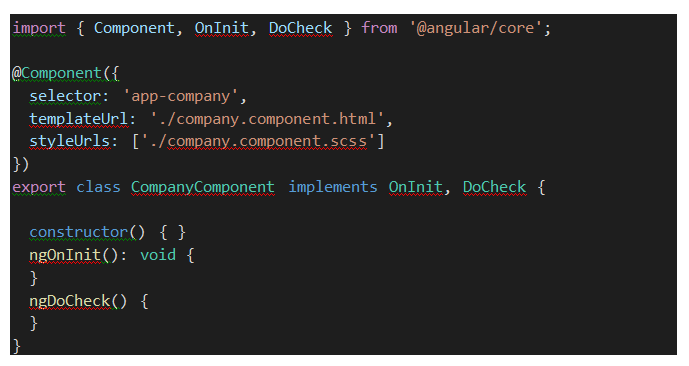
ngAfterContentInit() :
- When angular perform any external projection within the component’s view then this lifecycle event is executed. Before raising this event, also update the ContenChild and ContentChildren properties.
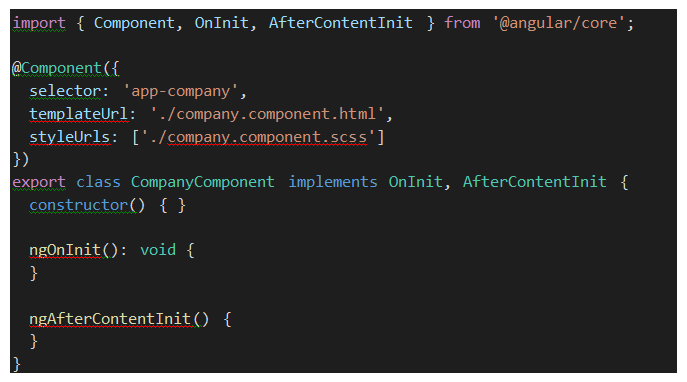
ngAfterContentChecked() :
- When the content of the component has been checked by the change detection mechanism this event is executed.
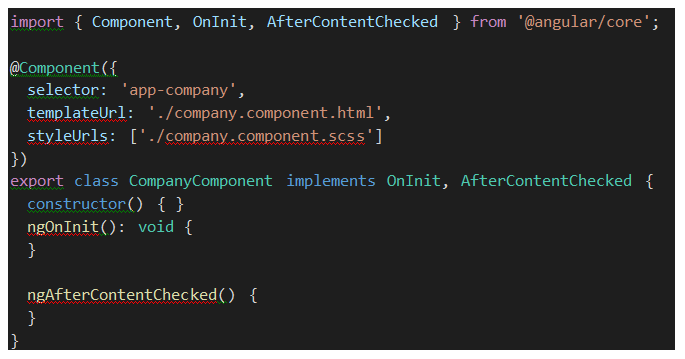
ngAfterViewInit() :
- When the component’s view has been initialized then the ngAfterViewInit() event is executed. This method is called after ngAfterContentChecked() event. This lifecycle event applies the only component.
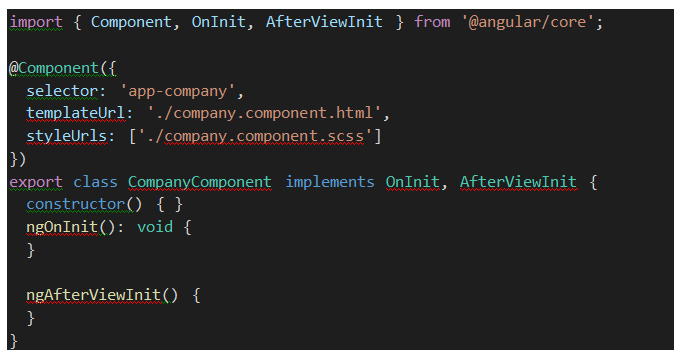
ngAfterViewChecked() :
- This event is called after ngAfterViewInit() event. When the view of the component has been changed by the change detection algorithm then this method is executed. This event is also called when data binding of the children’s directives has been changed. When the component waits for some value that is coming from the child component then this event is very useful.
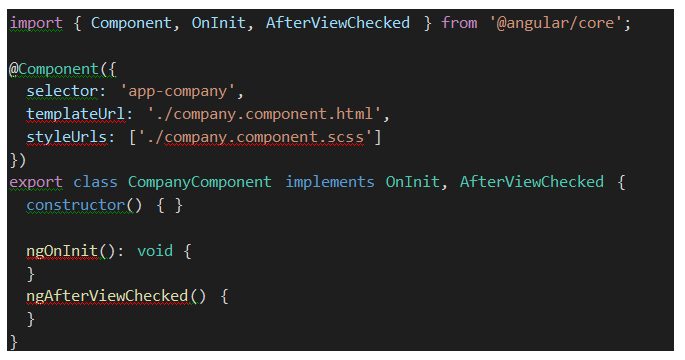
ngOnDestroy() :
- ngOnDestroy() event is executed just before angular destroys the component. This event is useful for detaching the event handlers to avoid memory leaks and unsubscribing the observable.
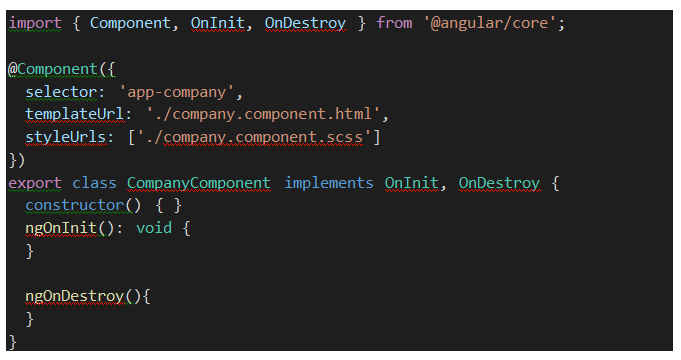
Conclusion:
In this blog, we have discussed the angular lifecycle and their events in which they occur in a lifecycle of a component. When you are creating an application, these lifecycle events are more important to control the components.