ReactJS: Elevating Your Web Development Skills to the Next Level
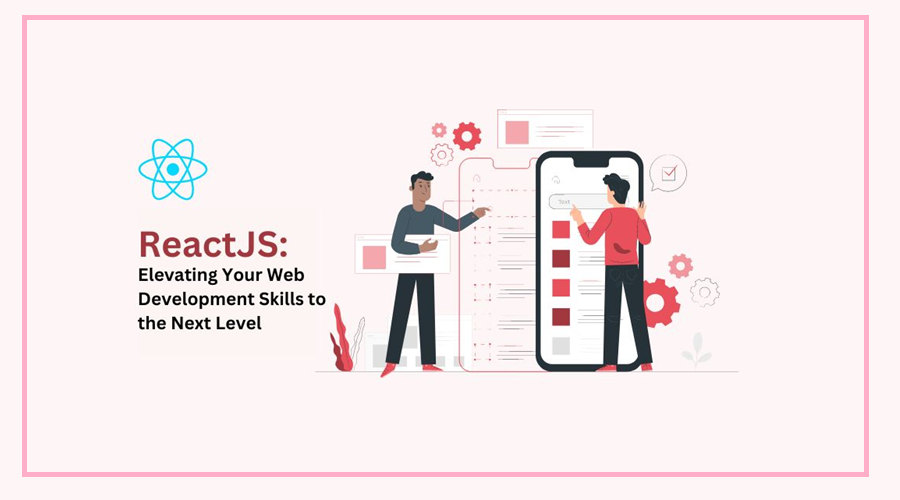
Are you tired of building websites that look like they were made in the ’90s? Do you want to take your web development skills to the next level and impress your clients and peers? Look no further than ReactJS, the JavaScript library that’s taking the web development world by storm.
ReactJS is not just a library, it’s a philosophy. It’s a way of thinking about web app development that emphasizes modularity, reusability, and efficiency. With ReactJS, you can build complex user interfaces with ease, thanks to its component-based architecture and declarative programming model.
But don’t just take our word for it. Here’s what one developer had to say about ReactJS: “ReactJS is like a superpower for web developers. It gives you the ability to build things that you couldn’t even imagine before. It’s like having a secret weapon in your toolkit.”
In this article, we’ll explore the history and key features of ReactJS, the advantages of using it in your web development projects, and best practices for building ReactJS apps. We’ll also cover advanced concepts like Higher-Order Components and React Hooks, as well as common mistakes to avoid.
So, put on your superhero cape and get ready to elevate your web development skills to the next level with ReactJS.
Understanding ReactJS
——————————–
History of ReactJS
ReactJS was created by Jordan Walke, a software engineer at Facebook, in 2011. It was initially used to build Facebook’s News Feed but was later open-sourced in 2013. Since then, it has gained immense popularity in the web development community and has been widely adopted by companies such as Netflix, Airbnb, and Dropbox.
How ReactJS works
ReactJS is a JavaScript library that uses a virtual DOM (Document Object Model) to efficiently render web pages. The virtual DOM is a lightweight copy of the actual DOM, which allows ReactJS to update only the necessary parts of the page when there are changes, resulting in faster rendering and improved performance.
ReactJS uses a component-based architecture, where each component represents a specific part of the user interface. These components can be reused across different parts of the website, making development faster and more efficient.
ReactJS also uses a declarative programming model, which means that developers can focus on describing what they want to achieve, rather than how to achieve it.
Key features of ReactJS
ReactJS has several key features that make it a popular choice for web development. One of the most important features is its component-based architecture, which allows developers to build reusable and modular components. This means that developers can save time by not having to rewrite code for similar parts of the website.
Another key feature of ReactJS is its ability to handle large and complex applications, thanks to its efficient rendering and performance. Additionally, ReactJS is easy to learn and use, with a large and supportive developer community. This community offers a wealth of resources, such as documentation, tutorials, and open-source projects, which can help developers learn and improve their ReactJS skills.
Advantages of Using ReactJS
——————————–
Faster rendering and performance
One of the key benefits of ReactJS is its efficient rendering and performance, which is due to its virtual DOM. The virtual DOM allows ReactJS to update only the necessary parts of the page when there are changes, resulting in faster rendering and improved performance. This makes ReactJS an excellent choice for large and complex applications that require fast and responsive user interfaces.
Reusability of components
Another major benefit of ReactJS is its component-based architecture, which allows developers to build reusable and modular components. This means that developers can save time by not having to rewrite code for similar parts of the website. This also makes it easier to maintain and update the website, as changes can be made to individual components rather than the entire website.
Easy to learn and use
ReactJS is known for its ease of use, thanks to its declarative programming model and large developer community. This means that developers can focus on describing what they want to achieve, rather than how to achieve it. Additionally, the large and supportive developer community offers a wealth of resources, such as documentation, tutorials, and open-source projects, which can help developers learn and improve their ReactJS skills.
Great developer community
Finally, ReactJS has a large and supportive developer community, which offers a wealth of resources and support for developers. This community provides documentation, tutorials, and open-source projects, which can help developers learn and improve their ReactJS skills. Additionally, the community is known for its helpfulness and willingness to share knowledge, making it a great place for developers to learn and collaborate.
Building a ReactJS App
——————————–
Setting up the environment
To build a ReactJS app, you need to have a development environment that includes a text editor, a web browser, and Node.js. Node.js is used to run the development server and manage dependencies. Once you have these tools installed, you can create a new ReactJS app using a tool like Create React App, which sets up the environment and provides a basic project structure.
Creating components
The key to building a ReactJS app is creating components. Components are reusable and independent parts of the user interface that can be combined to create the app. To create a component, you need to define its properties and methods, and then use them to render the component. ReactJS uses JSX, which is a syntax extension to JavaScript, to define components.
Adding interactivity with state
To make your ReactJS app interactive, you need to use state. State is an object that contains the data and properties of a component, and can be changed over time. By updating the state, you can change the behavior and appearance of a component. ReactJS provides several lifecycle methods, such as componentDidMount and componentDidUpdate, to manage state and handle events.
Using ReactJS libraries and tools
ReactJS has a large ecosystem of libraries and tools that can help you build better and more efficient apps. Some popular libraries include React Router, which provides routing for single-page applications, and Redux, which is a predictable state container for JavaScript apps. Additionally, there are many tools and extensions available for debugging, testing, and optimizing ReactJS apps.
Best Practices for Developing with ReactJS
——————————–
Writing reusable and maintainable code
To develop a ReactJS app that is maintainable and reusable, you need to follow best practices. This includes writing modular code, using meaningful names for variables and components, and separating concerns between components. Additionally, you should use comments and documentation to explain the code and its purpose.
Following the component-based architecture
One of the key benefits of ReactJS is its component-based architecture. To fully utilize this architecture, you should create components that are independent and reusable. Additionally, you should use props to pass data between components, and avoid directly modifying the state of other components.
Using ReactJS lifecycle methods effectively
ReactJS provides several lifecycle methods that can be used to manage state and handle events. To use these methods effectively, you should understand when each method is called, and what it is used for. Additionally, you should avoid using setState in the render method, as this can cause unnecessary re-renders and decrease performance.
Advanced ReactJS Concepts
——————————–
Higher-Order Components
Higher-Order Components (HOCs) are functions that take a component and return a new component with enhanced functionality. HOCs can be used to add features such as logging, caching, or authentication to components, without modifying their original behavior. This can make your code more modular and reusable.
React Hooks
React Hooks are a new feature in ReactJS that allow you to use state and other React features without writing a class. Hooks are functions that can be used in functional components to manage state, handle events, and perform other tasks. Some popular hooks include useState, which allows you to manage state, and useEffect, which allows you to handle side effects.
Context API
Context API is a feature in ReactJS that allows you to share data between components without using props. Context is an object that contains data that can be accessed by any component in the app. This can be useful for passing data such as user information or theme settings throughout the app.
Common Mistakes to Avoid
Not following best practices
One of the most common mistakes in ReactJS development is not following best practices. It’s important to write modular, maintainable, and reusable code that is easy to understand and update. Using meaningful names for components and variables, commenting and documenting the code, and separating concerns between components are all key practices to keep in mind.